Visual Studio Code on Linux
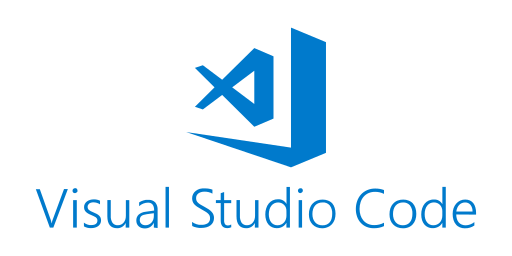
Microsoft declares that a new version of .Net and a new alternative dev tool Visual Studio Code will be available for multiple platforms, including Linux.
In this post, I will try to describe my Visual Studio Code usage experience. I will not describe .Net Code or DNX or Mono in details and focus on Visual Studio. I will use mono because .Net Core/DNX currently is incomplete and debugging using it is highly complicated under Linux. So I decided to use mono for now and switch to newer technologies later. I’m currently using Ubuntu 15.10 but all described things should work in the same way in 14.04 and any other Debian based on Linux.
First of all, you will need to set up the latest mono version. In the Canonical repository you always will find the old version, not sure why maybe some stability consideration but I’m not sure that Canonical guys test mono 🙂
Anyway to install the latest mono go to http://www.mono-project.com/docs/getting-started/install/linux/#debian-ubuntu-and-derivatives and follow the instructions about how to add the mono repository and install the latest version.
Next, let us install Visual Studio Code. You can download the latest version here https://code.visualstudio.com/ Downloaded file is just an archive with the application no installation process is required just unpack and start.
Also for some VS Code functionality, you will need DNX. Install it according to http://docs.asp.net/en/latest/getting-started/installing-on-linux.html or run the following commands to install DNX for mono:
curl -sSL https://raw.githubusercontent.com/aspnet/Home/dev/dnvminstall.sh | DNX_BRANCH=dev sh && source ~/.dnx/dnvm/dnvm.sh dnvm upgrade -r mono
Projects
There are no projects and solutions as it was in the usual Visual Studio. The general idea of visual studio code is that the project folder contains all project-related files and only project-related files. Also, all project-related files are programs in some human readable language (C#, JS, JSON, etc.) no more magic files with magic GUIDs. Thus you can only open the project folder not the project file with VS code and configure your project with any text editor, you can merge the project configuration with the merge tool, you can parse the project configuration with some automation tools, or do any other task based on documented and clear configuration files.
We still need project description files
If you open a folder with code and with no project files in VisualStudio, you will be able to use VS Code as a smart text editor and nothing more. However, any modern IDE should have code suggestions, code navigation, in-place error highlighting, debugging, and so on. Be sure VisualStudio Code supports these features and supports them on a higher level than VS 2015 Community Edition. But to enable all these features you have to explain VS some details about your code – create a project file.
What files can be used to configure the project?
Old project and solution files
VS Code supports *.sln
and project files. You cannot open the solution file but code parsing services will be able to locate and read solution/project files when you open the solution folder.
./**/project.json
A file named project.json is the main project configuration file. You can have several subprojects in your project and configure every project separately with project.json, in the case of .net every project file will produce an assembly. See the example below.
"configurations": { "Debug": { "compilationOptions": { "define": ["DEBUG", "TRACE"] } }, "Release": { "compilationOptions": { "define": ["RELEASE", "TRACE"], "optimize": true } } }, "frameworks": { "dnx451": { "frameworkAssemblies": { "System": "", "System.Runtime": "" } } }, "dependencies": { "Newtonsoft.Json": "8.0" }, "compile": "*/**/*.cs" }
This configuration file describes two configurations Debug and Release with different optimization settings and specific defined directives, defines one framework – dnx451 with used framework assemblies, and specifies required nuget packages in the “dpendencies” section. Compile section says that the project should include all *.cs files in all subdirectories (/**/
– means any subdir).
Please note that the project.json file is a part of the DNX build system and you have to install DNX to make it work.
Full specification of the project file can be found here https://github.com/aspnet/Home/wiki/Project.json-file.
./global.json
If you have several projects, you can group them together and explain VS Code that all project.json should be treaded as parts of some solution with global.json file.
One of my global.json
files looks like the following:
{ "projects": [ "Guardian.Common", "Guardian.Service", "Guardian.Module.BoilerMultiRoom", "Guardian.Module.RealtimeProvider", "Guardian.Module.Watering", "Guardian.Module.Update", "Guardian.Module.Video", "Guardian.Web.Common", "Guardian.Web" ] }
It just contains a list of all projects. You can find a description of the global.json file here http://docs.asp.net/en/latest/conceptual-overview/understanding-aspnet5-apps.html#the-global-json-file
./vscode/tasks.json
Here is an example of a tasks.json file of one of my real projects that have a mono back-end and typescript/html/less front-end.
{ "version": "0.1.0", "command": "gulp", "isShellCommand": true, "args": ["--no-color"], "tasks": [ { "taskName": "default", "isBuildCommand": true, "showOutput": "silent", "problemMatcher": ["$tsc", "$lessCompile", { "owner": "cs", "fileLocation": "relative", "pattern": { "regexp": "^\\S(.*)\\((\\d+),(\\d+)\\):.*(error|warning)(.*)$", "file": 1, "line": 2, "column": 3, "severity": 4, "message": 5 } }, { "owner": "general", "fileLocation": "relative", "pattern": { "regexp": "(error)(ed after)", "file": 1, "severity": 1, "message": 1 } }] }, { "taskName": "publish", "showOutput": "always", } ] }
As you can see, there are some global settings like command and args. Command in this file means what command should be executed to perform build actions. And yes, the command is global for all tasks. The command can be configured only once because with the command you should specify a build tool like msbuild, make or gulp in my case and every command is a target for build tools.
The actual command line will look like this.
The default task in my sample has isBuildCommand=true this means that VS Code should use this to build my project. You can execute the build task with Ctrl+Shift+B shortcut.
To execute other tasks you can press F1 and then type Run Task
followed by Enter. This will list all available tasks. Select one and press Enter to execute the task.
To parse the result of any task you can specify problem Matcher. Problem matcher is just a pattern to extract build errors, warnings, and any other messages. All extracted errors are shown as an error list in VS Code and are shown in-place in your code as it was in usual VS. You can use one of the existing problem matchers or define your own with a regular expression pattern.
Some of the available problem matchers
- $msCompile – Microsoft compiles (C# or C++)
- $lessCompile – Less files compiler
- $tsc – TypeScript compiler
- $gulp-tsc – TypeScript compiler implemented as a gulp task
Some notes about the single command for all tasks and build tools
At first, I was thinking as an experienced user of the usual Visual Studio Enterprise where we have a build and a lot of other “crunches” that allow us to automate tasks – “WTF same command for all tasks???”. But later I noted that usually we have to write another “build-crunches” to execute all these “crunches” on the build machine. In VS Code you are configuring build stages(targets) as tasks and should perform them through your build tool. That is a kind of DRY principle applied to build scripts. Write any task and you will be able to use it to build a machine.
VS Code tasks are a powerful tool that allows us to use any build system and integrate it with the code editor.
For more information about tasks see the following links.
https://code.visualstudio.com/docs/editor/tasks_appendix
https://code.visualstudio.com/Docs/editor/tasks
./vscode/launch.json
lunch.json describes how to execute the end debug the application when you press F5 key. Here is an example from one of my projects:
{ "version": "0.2.0", "configurations": [ { "name": "Launch", "type": "mono", "request": "launch", "program": "./publish/Guardian.Service.exe", "args": [], "cwd": "./publish/", "env": {} }, { "name": "Attach", "type": "mono", "request": "attach", "address": "localhost", "port": 5858 } ] }
Currently VS Code supports only two lunch configurations: “Launch” and “Attach” to start debugging and attach to the already started processes. You can specify the type of application, currently only “mono” or “node” are supported under Linux, and specify a program to start or host/port to attach a debugger to.
Unfortunately, there is no way to debug .Net Core in VS Code under Linux now. Hope to see it in the nearest future. The most significant problem here is .pdb/.mdb files problem. VS Code for Linux supports mono code mapping files and .Net Core supports usual pdb files. Hope it is just a question of time as far as Windows VS Code can debug .Net applications.
Ensure that the project description is parsed correctly
When you open a folder with just project.json file, VS Code automatically parses this file and enables functionality like suggestions and code navigation. In this case, you will see the “Running” status bar indicating that VS Code is parsing the project file.
When the project is parsed successfully you will see a status like the following.
By clicking on the project name in the status bar you can select the project file manually.
In some cases, VS Code will not be able to select a project files. In such case, it will show “Select project” green text in the status bar and you will have to select the project file manually.
When the project file is parsed by VS you will be able to use functionality like code navigation, suggestions, etc.
Built-in GIT support
VS Code has a built-in git client. That allows you to perform simple git tasks like push/pull, rebase, commit, select files for commit, revert specific files, and so on. More complex tasks like view history and merge conflicts look not very nice in the current version of VS Code and are more likely you will use some external tools for these tasks.
Read more at https://code.visualstudio.com/Docs/editor/versioncontrol
Other languages and technology support
Visual studio code supports a lot of languages except C# and support of some languages is even better than in VS Enterprise
Features | Languages |
---|---|
Syntax coloring, bracket matching | Batch, C++, Clojure, Coffee Script, Dockerfile, F#, Go, Jade, Java, HandleBars, Ini, Lua, Makefile, Objective-C, Perl, PowerShell, Python, R, Razor, Ruby, Rust, SQL, Visual Basic, XML |
Snippets | Groovy, Markdown, PHP, Swift |
IntelliSense, linting, outline | CSS, HTML, JavaScript, JSON, Less, Sass |
Refactoring, find all references | TypeScript, C# |
Summary
- You can use Visual Studio Code write, refactor and debug .Net/Mono (C#) code under any OS
- Support of other languages makes VS Code a highly efficient tool for mixed projects with TypeScript, Less and C# code for example.
- Support of custom build tools adds more value to VS Code as a tool for complex mixed projects
- All project configurations are human readable JSON that can be easily maintained
- VS Code has built in git support that solves 90% of tasks
- Usage of VS Code requires another point of view on development – usage of easy to understand config files instead of wizards
See my next post for a sample project.
Some useful links
Debugging in Visual Studio Code
Version Control in Visual Studio Code