Explicit call to RequireJS in TypeScript
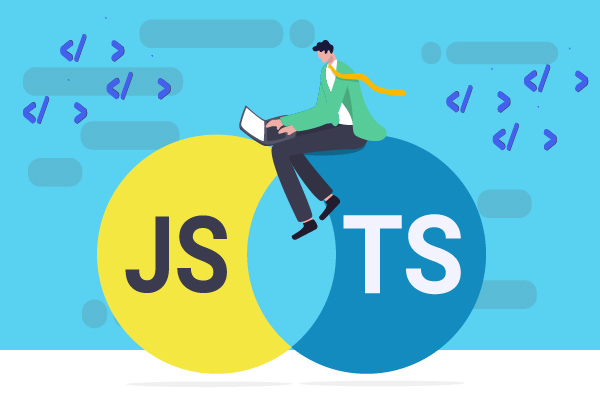
I’m working on a mobile application with Apache Cordova (http://cordova.apache.org
) technology. And one of the tasks was to load a JSON localization file from the content folder. The first idea and actually the most correct (as for me) is to load the file with RequireJS text plugin (https://github.com/requirejs/text
).
The plugin allows loading text file in the same way as usual modules and do not evaluate the content, but return it as a string. So you just specify something like the following.
require(["some/module", "text!some/module.html"], function(module, html, css) { //the html variable will be the text //of the some/module.html file } );
When using TypeScript we can write
import html = require("text!some/module.html"); document.body.innerHTML = html;
And this will give us the following JS code of the module (In case of AMD mode in compiler)
define(["require", "exports", "text!some/module.html"], function (require, exports, html) { document.body.innerHTML = html; });
Unfortunately, it’s not enough in case of localization, because we have to load a specific html file for a specific locale (text!/locale/en/module.html). And we have to select the path dynamically depending on the selected locale. In JS we can write the following.
define(["require", "exports","someService"], function (require, exports, someService) { var locale = someService.getLocale(); require(["text!/" + locale + "/en/module.html"], function(html){ document.body.innerHTML = html; }); });
First, it was not absolutely clear to me how to do it in TypeScript. There is no explicit import of RequireJS in typescript, and require is a keyword used as part of a module declaration. I’ve tried to find some description for my case, but without any success. Fortunately, the solution is much simpler than I thought:
1. You should add requre.d.ts typing to your project (or to the compiler command line)
2. Then just write the following TypeScript
var locale = someService.getLocale(); require(["text!/" + locale + "/en/module.html"], html => document.body.innerHTML = html);
And this will give exactly the same code as in JS sample above. And you can ignore the editor warning about keyword require, just compile the project and you will get no errors. Please note that I’ve tested this with TypeScript 1.4 compiler and with MS Visual Studio 2013. And don’t forget to use an array of strings as the first argument of require, but not string as in another require syntax.
If you have any other ideas how to make it work please add comments.